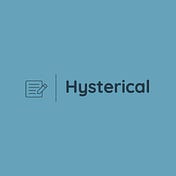
Hysterical
Sharing insights into Technology topics and Tech Careers. Thoughts shared here are personal and not affiliated with any other entity. All Rights Reserved.
Sharing insights into Technology topics and Tech Careers. Thoughts shared here are personal and not affiliated with any other entity. All Rights Reserved.